In the Xerox Palo Alto Research Center between 1973 and 1976, Larry Tesler implemented the first version of what will be later known as the Copy-Paste tool. This tool is indeed very handy especially in graphical user interfaces and I guess we no longer can still live without it, but it also can be pretty convenient in text-based interface (as the terminal) without requiring the mouse.
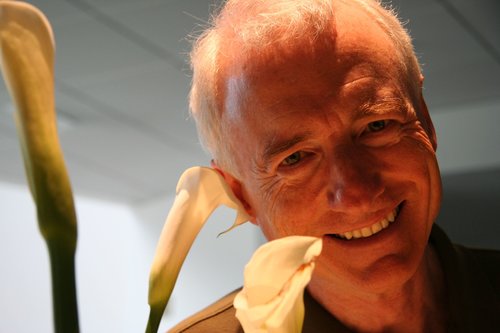
Larry Tesler, 2007. Picture under CC License. Source Wikimedia
Two commands exist in Apple’s OS and they are pbcopy and pbpaste.
The power of these two commands comes from the fact that we can use them with redirections and pipes. Here are some examples:
ps -A | pbcopy
ls -la | pbcopy
cat ./file.txt | pbcopy
or simpler:
pbcopy < ./file.txt
And then you will be able to paste the content of your clipboard like this:
pbpaste >> ./anotherfile.txt
Quite helpful isn’t it ?